Setting up your environment ๐ณ
1
2
mkdir contensis-dot-net
cd contensis-dot-net
Using a .Net template ๐๏ธ
Creating our application ๐ป
1
2
3
4
5
6
7
8
9
10
11
12
13
14
using System;
using Zengenti.Contensis.Management;
using DotNetEnv;
namespace CreateEntries
{
class Program
{
static void Main()
{
}
}
}
1
2
dotnet add package Zengenti.Contensis.Management
dotnet add package DotNetEnv
Setting up the client ๐งฐ
1
2
3
4
5
6
7
8
9
// Load the .env file into environment variables
DotNetEnv.Env.Load();
// Client Initlisation
var client = ManagementClient.Create(
rootUrl: string.Format("https://api-{0}.cloud.contensis.com", DotNetEnv.Env.GetString("ALIAS")),
clientId: DotNetEnv.Env.GetString("CLIENT_ID"),
sharedSecret: DotNetEnv.Env.GetString("CLIENT_SECRET")
);
Creating our API key ๐
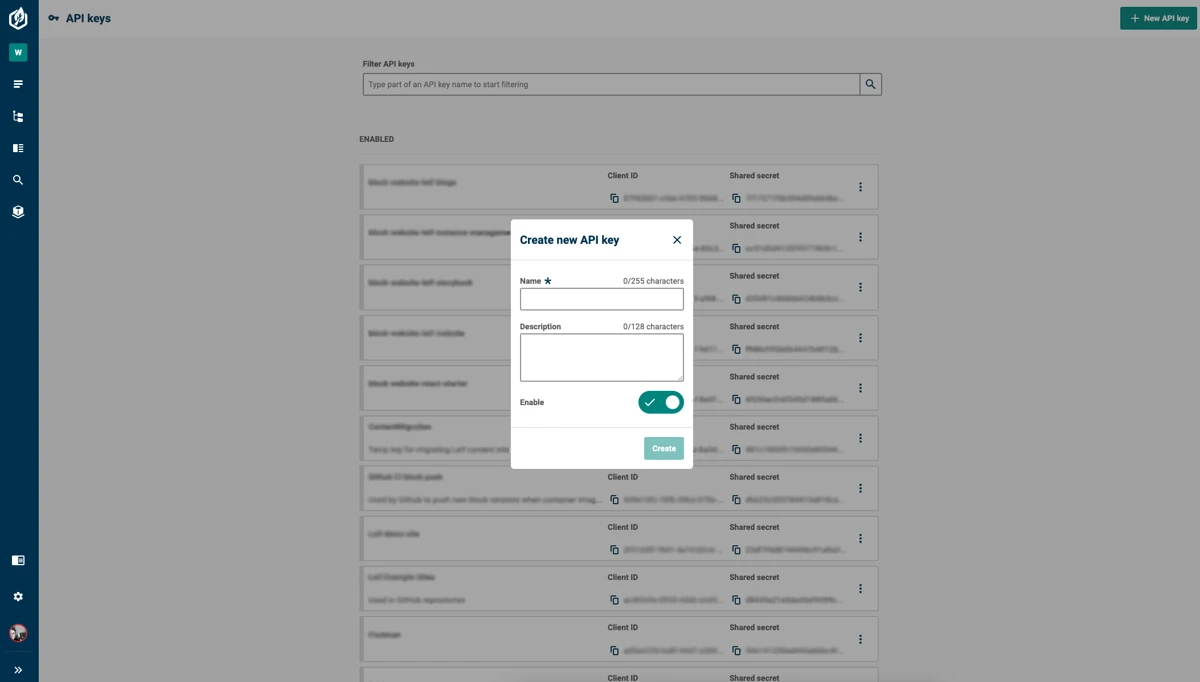
1
2
3
CLIENT_ID= {your client ID}
CLIENT_SECRET= {your shared secret}
Configuring your PROJECT_API_ID and ALIAS โ๏ธ
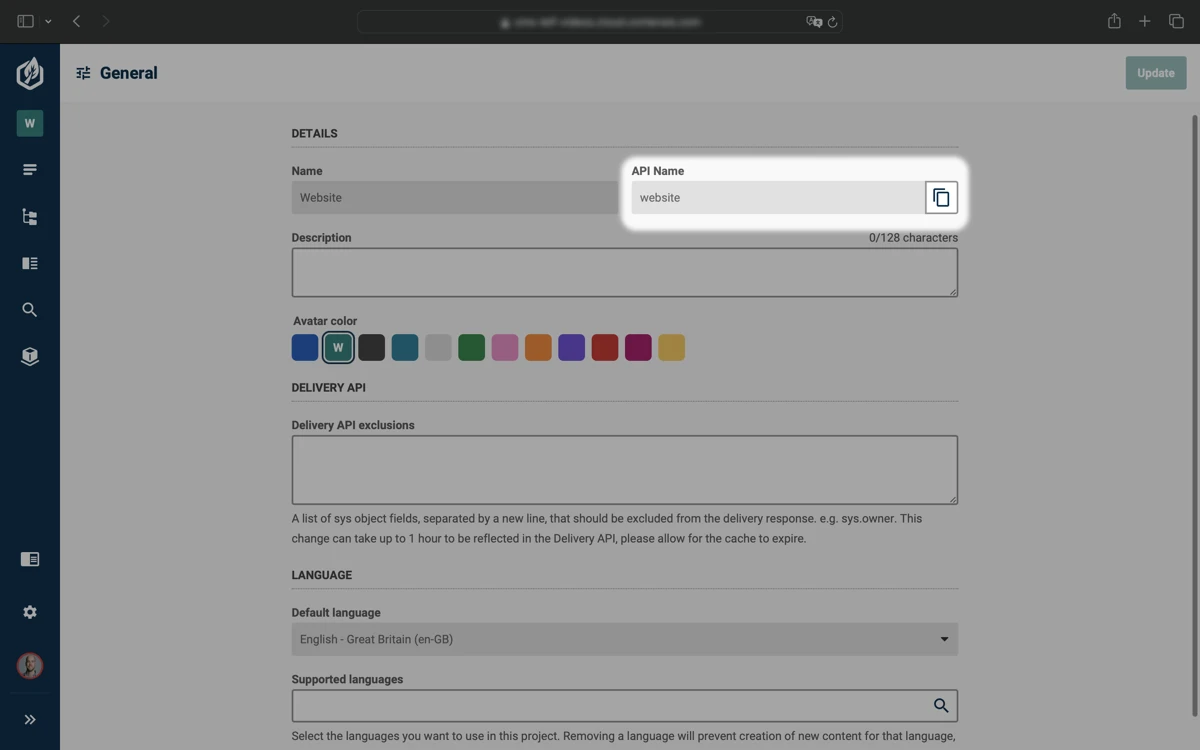
1
2
3
PROJECT_API_ID= {yourProjectId}
ALIAS= {yourAlias}
Setting up API key roles and permissions ๐ค
Creating our first Content Type ๐จ
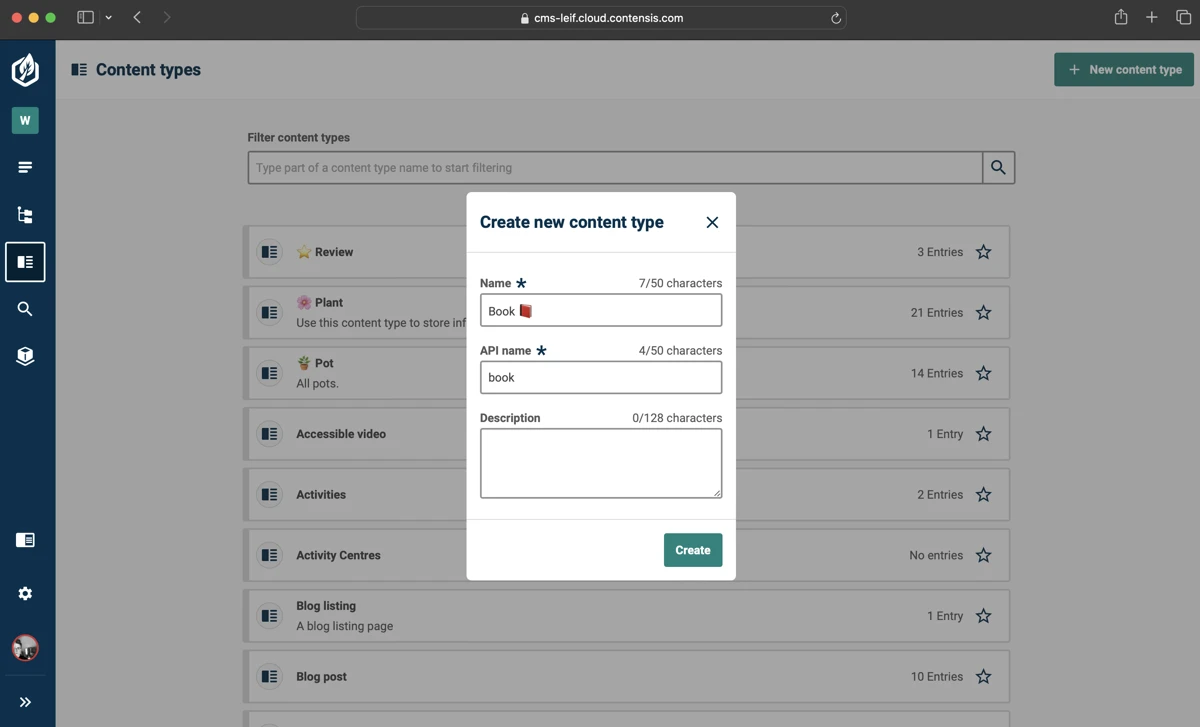
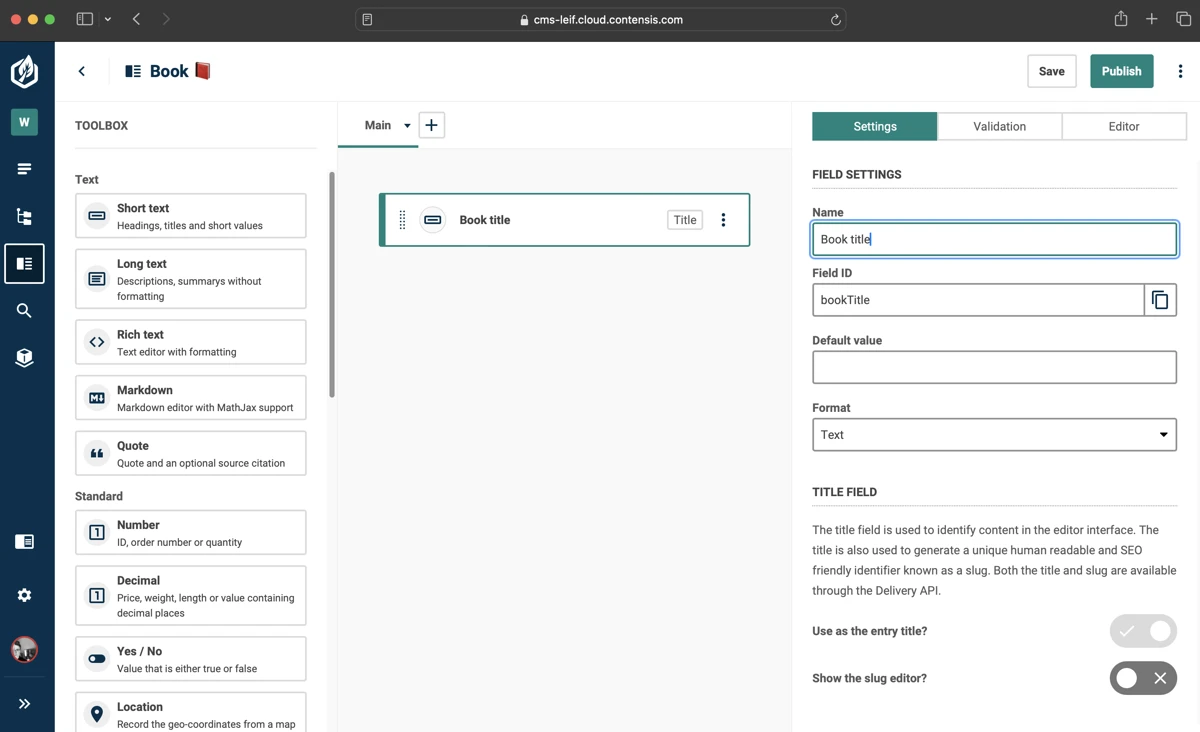
Creating our first Entry ๐
1
2
// Retrieve Project
var myProject = client.Projects.Get(DotNetEnv.Env.GetString("PROJECT_API_ID"));
1
2
// Create Entry
var entryBook = myProject.Entries.New("book");
1
2
// Set Fields
entryBook.Set("bookTitle", "How not to kill your houseplants ๐ฑ");
1
2
3
4
5
6
7
8
9
10
11
// Save Entry
try
{
entryBook.Save();
Console.WriteLine("Successfully Created Entry!");
}
catch (Exception ex)
{
Console.WriteLine("An unexpected error occurred: " + ex.Message);
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
using System;
using Zengenti.Contensis.Management;
using DotNetEnv;
namespace CreateEntries
{
class Program
{
static void Main()
{
// Load the .env file into environment variables
DotNetEnv.Env.Load();
// Client Initlisation
var client = ManagementClient.Create(
rootUrl: string.Format("https://api-{0}.cloud.contensis.com", DotNetEnv.Env.GetString("ALIAS")),
clientId: DotNetEnv.Env.GetString("CLIENT_ID"),
sharedSecret: DotNetEnv.Env.GetString("CLIENT_SECRET")
);
// Retrieve Project
var myProject = client.Projects.Get(DotNetEnv.Env.GetString("PROJECT_API_ID"));
// Create Entry
var entryBook = myProject.Entries.New("book");
// Set Fields
entryBook.Set("bookTitle", "How not to kill your houseplants ๐ฑ");
// Save Entry
try
{
entryBook.Save();
Console.WriteLine("Successfully Created Entry!");
}
catch (Exception ex)
{
Console.WriteLine("An unexpected error occurred: " + ex.Message);
}
}
}
}