Using Contensis with .Net Razor Pages
Log in to add to favouritesThis step-by-step guide will take you through getting your entries from Contensis and displaying them using the Delivery API and a simple .Net Razor Pages app.
โ๏ธ Prerequisites
- Git
- Basic command line interface knowledge
- A GitHub account (New to GitHub? Sign up here)
- Either Visual Studio or VS Code
Visual Studio
- Visual Studio 2022 with the ASP.NET and web development workload.
VS Code
We'll start by setting up our demo project, which is a simple .NET blog application. This app will pull in data from our demo Leif project using Contensis.
Fork the Razor Pages Leif example ๐ด
First, we need to fork the Razor Pages Leif example on GitHub to create our own copy of the repo. To do this:
- Log into your GitHub account.
- Go to https://github.com/contensis/razor-page-leif-example and fork the repo.
Cloning the repository ๐ค
Now we have our own fork of the repository, we need to clone it to create a copy of the fork on our local machine. There are two ways to do this โ using SSH or HTTPS. If you have already set up SSH keys for your GitHub account, then it's easier to use SSH. If you haven't set up SSH keys, you can use HTTPS instead.
Clone the repository using SSH
- First, open up a terminal and
cd
into your local development folder. - Now clone the repository using SSH with the following command:
git clone git@github.com:{your-username}/razor-page-leif-example.git
- Then navigate to the project directory:
cd razor-page-leif-example
cd RazorPageLeifExample
Clone the repository using HTTPS
If you haven't set up SSH keys for your GitHub account, you can use HTTPS instead of SSH to clone repositories. Use the following steps to configure Git to use HTTPS.
- Depending on your operating system, open Terminal (on macOS and Linux) or Command Prompt (on Windows).
- Enter the following commands to configure Git with your GitHub credentials:
git config --global user.name "Your GitHub Username"
git config --global user.email "your.email@example.com"
- Now enter the following command to clone the repository using HTTPS:
git clone https://github.com/{your-username}/razor-page-leif-example.git
- Then navigate to the project directory:
cd razor-page-leif-example
cd RazorPageLeifExample
Running the Application ๐ฅณ
Now we've successfully cloned the repo and are in the correct folder, we can run the app locally on our machine. To run your app locally, enter the following command in your terminal:
dotnet watch
This will start the server. You can then access the application by visiting http://localhost:3000
in your web browser.
The application should look something like this:
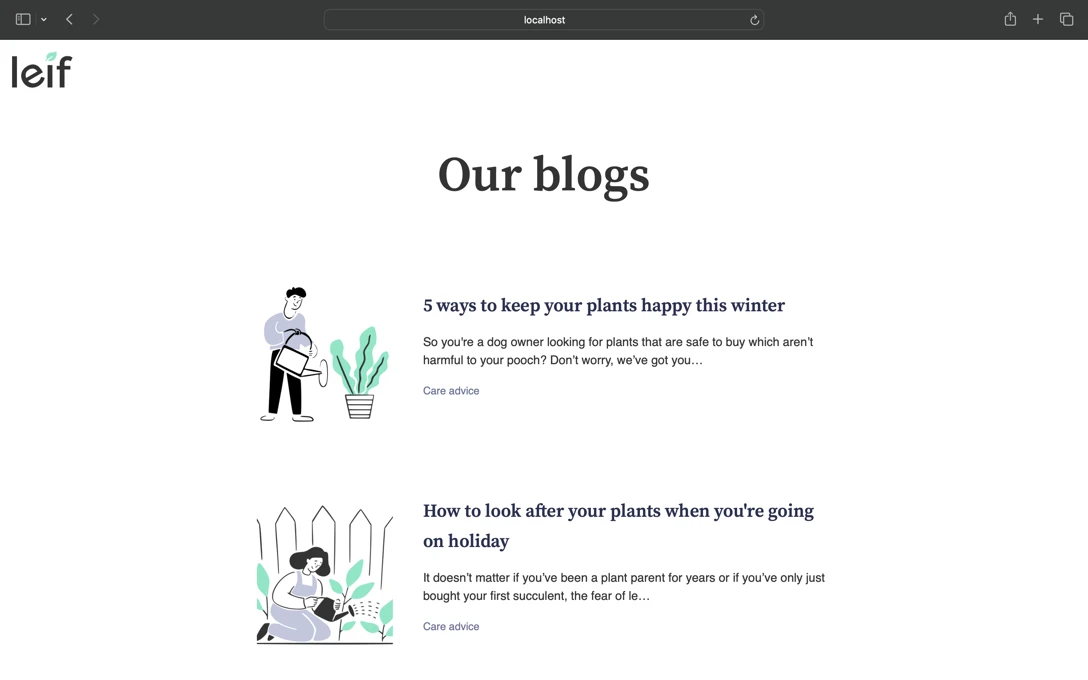
If you get any errors, make sure the following are installed on your machine:
Using your own content ๐
At the moment the application is using data from our public demo environment. To start using your own data, you'll need to connect your own Contensis environment. Follow these steps to connect a free trial environment:
- Create an account with Contensis or log in.
- Navigate to your account dashboard and launch your free trial environment. (If you don't have a free trial, you can create one here).
- The first thing we need to do is update the
CONTENSIS_CLIENT_ID
andCONTENSIS_CLIENT_SECRET
in the.env
file with credentials from our own API key. Go to Settings > API Keys in your Contensis project. - Press the New API Key button in the top-right corner to create a new API key.
- Copy the
CONTENSIS_CLIENT_ID
andCONTENSIS_CLIENT_SECRET
from your API key into the.env
file.
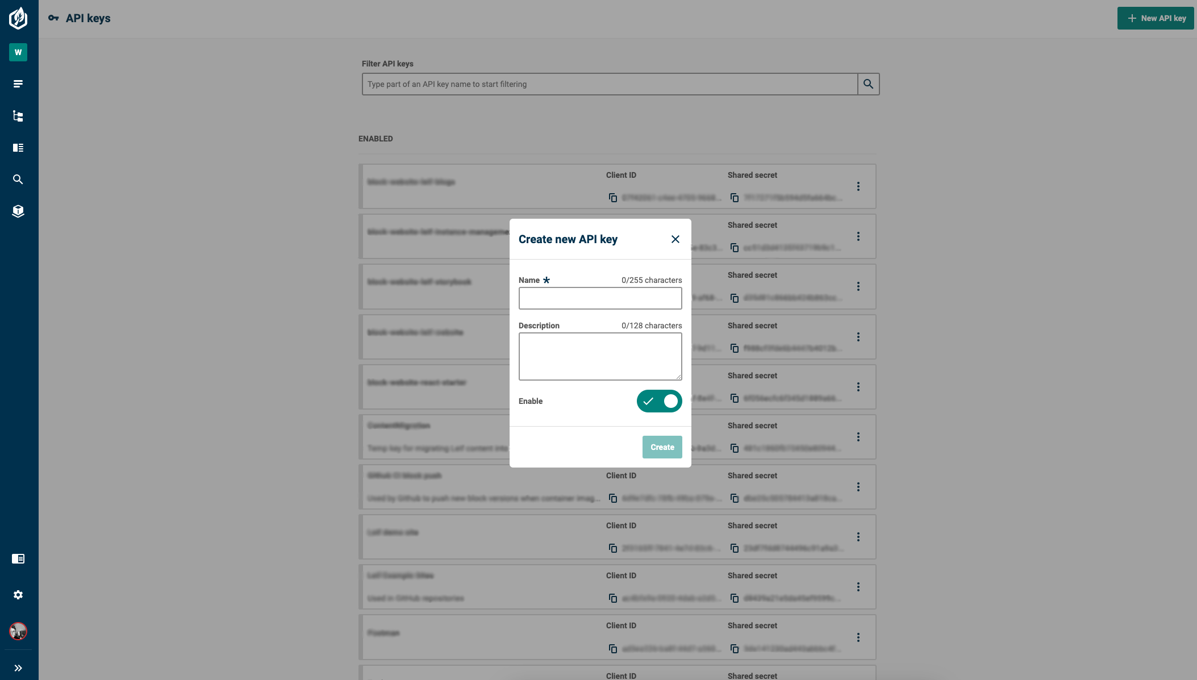
- You now need to replace the
PROJECT_API_ID
in the.env
file with the API ID from your own project. In your Contensis project, go to Settings > General and copy the API Name field.
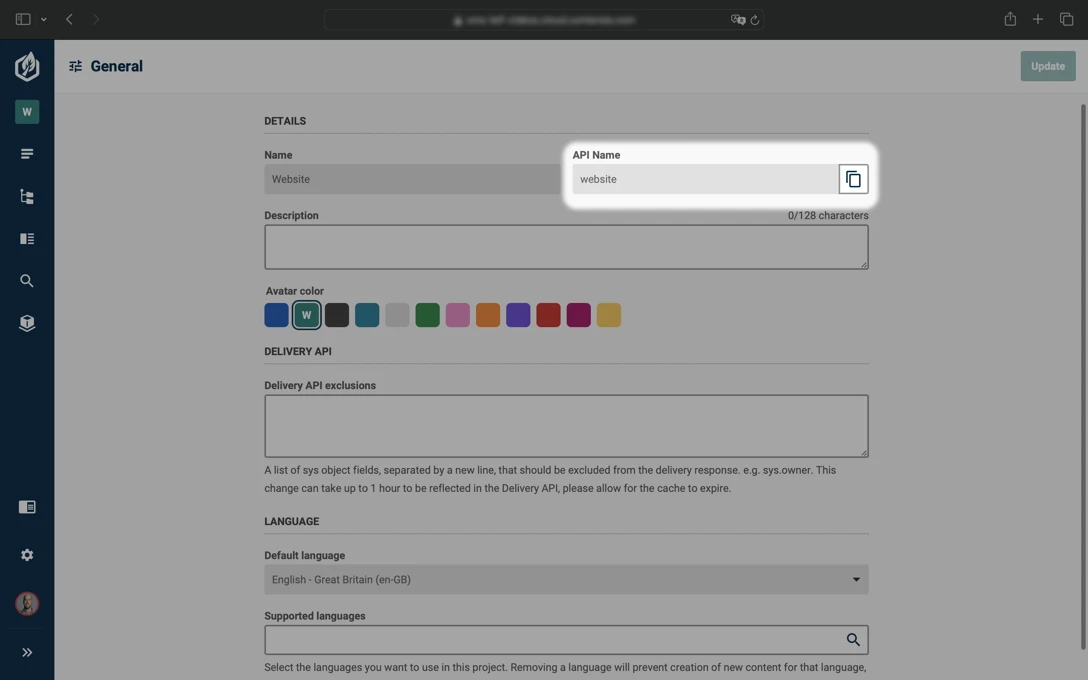
- Open the
.env
file in your IDE again and replace thePROJECT_API_ID
with the API ID from your Contensis project. - Finally, determine your
ALIAS
by examining your CMS URL, for instance, cms-leif.cloud.contensis.com. Extract the part that follows cms-. In this example, theALIAS
would be 'leif'.
That's it, you're all set up! ๐